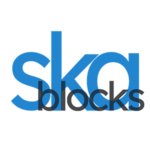
ska-blocks
What is ska-blocks? How to add Tailwind classes to WordPress blocks? What are selectors? How do presets work?
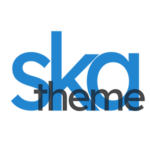
ska-theme
How to use ska-theme? What templates does it come with? What modules are included? How to make UI elements? What integrations are available?